
How to use Python Tkinter MassegeBox to alert users on wrong calculations?
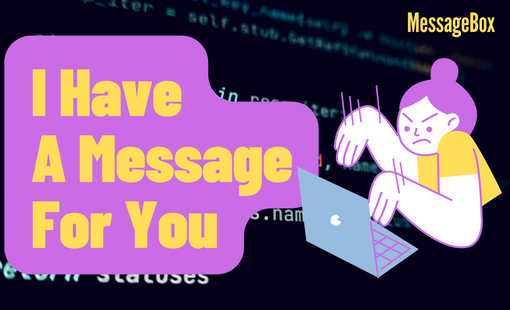
Post by Amina Delali, November 8th, 2022
Description
A message box is that box that appears when an app wants you to know something. It could be just an informative message, or an indication of a warning or an error. The app could also ask you to confirm something by clicking on a specific button. In Python, particularly Tkinter, one of the ways of creating these Message Boxes is to use the TK MessageBox widget.
In this code, the user enter two number values on two Entry TK widgets, then click on one of four Buttons. Each button corresponds to an arithmetic operation. Depending on the results and the given values, the user may see 3 types of MessageBox widgets:
- A warning message box, that shows up when the user doesn't give a proper number values.
- An error message box, when the operation is a division, and the second number is a zero.
- An information message box, that displays the result of the calculation.
The functions used in the code are:
- 4 functions that correspond to the 4 arithmetic operations:
- divide: the division is in a try except block. If the second operand value of the division is a zero, the Error MessageBox will be displayed from the except block
- multiply: the multiplication operation
- add: the addition operation
- subtract: the subtraction operation
- the function setResult:
- It will display the result of an operation; given as a parameter, in the corresponding Entry widget
- Also will indicate the result by displaying an Informative MessageBox.
- The getValues function, that will get the values from the Entry widgets as text then convert them into float, after that, it will return the resulting values:
- The conversion is made in a try except block. If the text does not correspond to a valid number, it will display a Warning MessageBox created in the except blok. Then set the result to zero.
- After converting the two text values, the results are returned to the calling functions.
To get an idea of all the different MessageBoxes that you can create, you can check this link, or watch the video below 🙂.
The Code
from tkinter import *
from tkinter import messagebox
from turtle import right
main_window=Tk()
main_window.title("This a messagebox example")
main_window.geometry('350x250+400+100')
main_window.configure(bg="#8800FF")
number1L= Label(main_window,text="Number 1",bg="white", fg="#8800FF")
number1L.grid(row=0, column=0,pady=20, padx=20)
number1 = Entry(main_window,width = 30)
number1.grid(row=0, column=1,pady=20, padx=20)
number2L = Label(main_window,text="Number 2",bg="white", fg="#8800FF")
number2L.grid(row=1, column=0,pady=20, padx=20)
number2 = Entry(main_window,width = 30)
number2.grid(row=1, column=1,pady=20, padx=20)
def getValues():
try:
val1=float(number1.get())
except ValueError:
messagebox.showwarning("No Number", "You didn't give a number for the first value")
val1=0
try:
val2=float(number2.get())
except ValueError:
messagebox.showwarning("No Number", "You didn't give a number for the second value")
val2=0
return [val1,val2]
def setResult(res):
result.delete(0,END)
result.insert(0,str(res))
messagebox.showinfo("Calculation Done", "The result is: "+ str(res))
def divide():
val1,val2=getValues()
try:
res= val1/val2
except ZeroDivisionError:
messagebox.showerror("0 Division Problem", "You are dividing by 0")
res=0
setResult(res)
def multiply():
val1,val2=getValues()
res= val1*val2
setResult(res)
def add():
val1,val2=getValues()
res= val1+val2
setResult(res)
def subtract():
val1,val2=getValues()
res= val1-val2
setResult(res)
operationsF = Frame(main_window)
operationsF.grid(row=2, column=1)
divide=Button(operationsF, text="/",bg="#00FA92",command=divide)
divide.pack(side="right")
multiply=Button(operationsF, text="*",bg="#00FA92",command=multiply)
multiply.pack(side="right")
add=Button(operationsF, text="+",bg="#00FA92",command=add)
add.pack(side="right")
subtract=Button(operationsF, text="-",bg="#00FA92",command=subtract)
subtract.pack(side="right")
resultL = Label(main_window,text="Result",bg="#8800FF", fg="white")
resultL.grid(row=3, column=0,pady=20, padx=20)
result = Entry(main_window,width = 30)
result.grid(row=3, column=1,pady=20, padx=20)
main_window.mainloop()
The Output
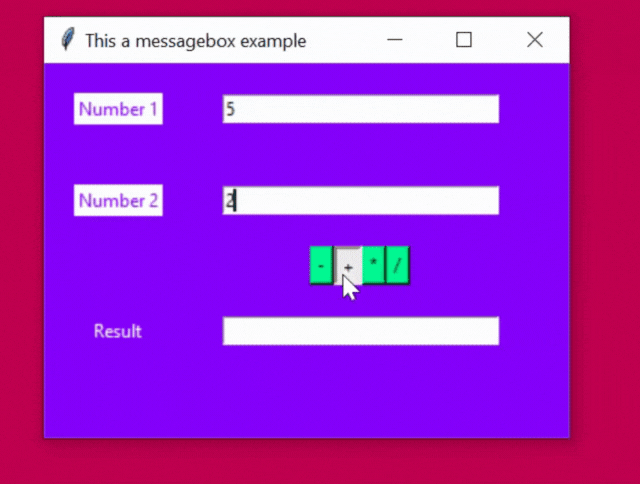
Something to say?
If you want to add something about this post, please feel free to do it by commenting below 🙂.