
How to Use The Entry widget for Passwords in Python?
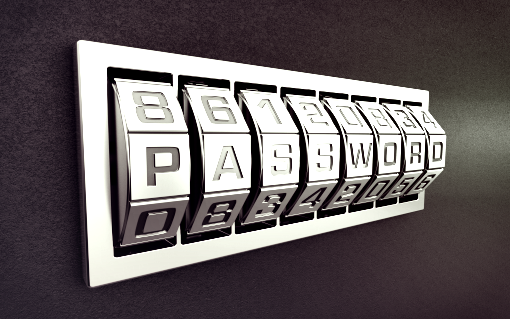
Post by Amina Delali, August 16th, 2022
What is the Entry Widget in Python ?
The entry widget is an input widget that enables the user to interact with your application. It allows him to enter one line of text. However, you can not specify the type of input required by the user. For example, there is no option to tell the widget to accept only digits. Nevertheless, you can implement by yourself events functions that can restrict the type of input.
You can also define functions to check the data after the user entered his text. This is what we are going to do in this post.
Description of the code: Verify Passwords
In the following code, 2 Entry widgets used to accept passwords indicated by 2 corresponding title labels (they will be in a frame to use different layout managers), one button to check the input, and a label to display the result:
- You enter your password in the first widget.
- Then, you enter the same password in the second widget.
- The widget is set to show stars instead of letters when typing the text.
- You click on the button "Validate". If the passwords are the same, you see a message displayed in a label indicating that the passwords are identical. If it is not the case, an error message is displayed. If one of the passwords entries are empty, a message will indicate that in the same previous label.
The Code
from cProfile import label
from email import message
from tkinter import *
main_window = Tk()
main_window.title("Entry Widget TK")
main_window.geometry("400x400+400+150")
main_window.configure(bg="#f4ff00")
framePasswords= Frame(main_window,bg="#f4ff00")
framePasswords.pack(pady=10,fill="x")
labFirstPassword = Label(framePasswords,text="Password",bg="#f4ff00")
labFirstPassword.grid(row=0,column=1,pady=10)
firstPassword = Entry(framePasswords,show="*",width = 30)
firstPassword.grid(row=0,column=2,pady=10)
labSecondPassword = Label(framePasswords,text="Confirm Password",bg="#f4ff00")
labSecondPassword.grid(row=1,column=1,pady=10)
secondPassword = Entry(framePasswords,show="*",width = 30)
secondPassword.grid(row=1,column=2,pady=10)
messageLabel = Label(main_window, text="",bg="#f4ff00", font=("Arial",18))
messageLabel.pack(pady=10)
def checkPasswords():
messageLabel.configure(text="")
text1= firstPassword.get()
text2= secondPassword.get()
if not text1 or not text2:
messageLabel.configure(text="Passwords Must not be empty", fg="red")
return
if text1 == text2:
messageLabel.configure(text="The Passwords are Equal",fg="green")
else:
messageLabel.configure(text="The Passwords Are Different\nThey must be Equal",fg="red")
validateButton = Button(main_window,text="Validate",command=checkPasswords,bg="#8800FF",fg="white")
validateButton.pack(pady=20)
main_window.mainloop()
The Output
Something to say?
If you want to add something about this post, please feel free to do it by commenting below 🙂.