
Use the Scale TK widget to change the Size of a Text
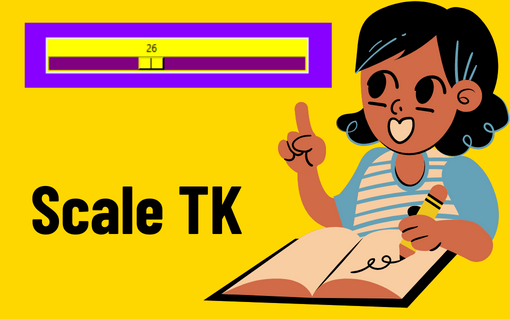
Post by Amina Delali, December 19th, 2022
Description
In this post, I describe how to use the Scale TK widget of Python Tkinter to change the size of a displayed Text. The text is displayed with a Label widget.
The Scale widget can be used to select a value ranging between a minimum and a maximum value. By sliding a handle on the widget, the actual associated value will change, according to different parameters:
- from_: defines the value of one end of the scale range. In our code, the value is equal to 12
- to: defines the value of the other end of the scale range. In our case, the value is equal to 48
- resolution:defines the value of the increment between two consecutive values from the scale. In our case, it is set to the default value, which is 1.0
To change automatically the size of the text when sliding the scale:
- Set the command attribute of the Scale widget to the function updateText
- The function updateText takes one parameter, that will be the value associated with the widget; corresponding to the position of handle of the scale widget, and it changes the size of the font attribute associated with the Label widget
- The function will also change the text of the Label in question
- When the Label is created, its font size is set to 12. In our case, it is the minimum value of the scale . The text is also set accordingly: "Size = 12"
The widgets used in this code are:
- Label TK: this is the widget used to display a text. Changing the size of its font attribute, will change the size of its text.
- Scale TK: this is the widget used to change the value of the size of the label's font.
The Code
from tkinter import *
main_window=Tk()
main_window.title("Scale TK")
main_window.geometry('400x450+400+100')
main_window.configure(bg="#8800FF")
label = Label(main_window,bg="#8800FF",fg="yellow",
font=("Arial",12,"bold"),
text= "Size = 12")
label.pack(pady=80)
def updateText(val):
label.config(font = ("Arial",val,"bold"))
label.config(text="Size = "+str(val))
var = IntVar()
scale = Scale( main_window, variable = var , orient=HORIZONTAL,
troughcolor="purple",relief=FLAT,bg="yellow",
from_=12,to=48,length=300, command=updateText)
scale.pack(pady=20)
main_window.mainloop()
The Output
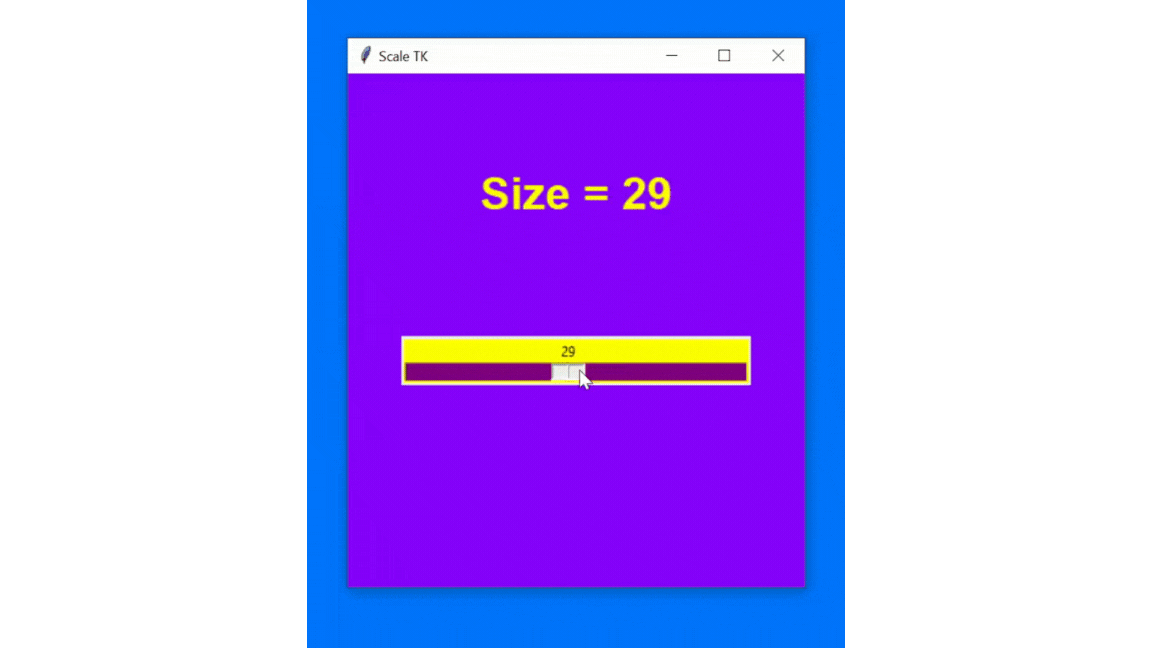
Something to say?
If you want to add something about this post, please feel free to do it by commenting below 🙂.