
Create a Simple Time Calculator (TK)
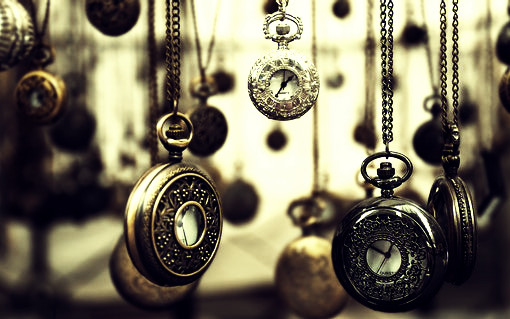
Post by Amina Delali, December 30th, 2021
Description
In this code I create a simple Time Calculator using Python Tkinter TK Entry widgets, Frames, Labels and buttons. You entre two values of time duration in seconds, minutes, hours, and days. Then you click on the equal button to get the the sum of the two values. The sum will also be displayed in seconds, minutes, hours, and days.
The Code
from tkinter import *
main_window = Tk()
main_window.title("Time Calculation")
main_window.geometry("700x200+200+300")
def getValues(theList):
val=[]
for i in theList:
if i.get():
val.append(int(i.get()))
else:
val.append(0)
return val
def calcTime(theList):
seconds = theList[3]
minutes = theList[2]*60
hours = theList[1]*60*60
days = theList[0]*24*60*60
return seconds+minutes+hours+days
def convertTime(timeV):
timeL=[]
timeL.append(timeV//86400)
rem = timeV % 86400
timeL.append(rem//3600)
rem = rem % 3600
timeL.append(rem//60)
rem = rem % 60
timeL.append(rem)
return timeL
def fillEntries(values,theList):
for i in range(4):
theList[i].delete(0,"end")
theList[i].insert(0,str(values[i]))
first =[]
second = []
third = []
theFrame = Frame(main_window,width=700,height=200)
theFrame.pack(expand=FALSE)
frame1 = Frame(theFrame)
frame1.pack(side=LEFT,pady=20)
frame2 = Frame(theFrame)
frame2.pack(side=RIGHT,pady=20)
frames=[]
for i in range(0,6):
frames.append(Frame(frame2))
frames[i].pack()
titles=[]
titlesT=["Days","Hours","Minutes","Seconds"]
for i in range(0,4):
titles.append(Label(frames[0],text=titlesT[i]))
first.append(Entry(frames[0]))
second.append(Entry(frames[2]))
third.append(Entry(frames[4],bg="lightgray",fg="green",font=("times",10,"bold")))
titles[i].grid(row=0,column=i)
first[i].grid(row=1,column=i)
second[i].grid(row=0,column=i)
third[i].grid(row=0,column=i)
plusLabel=StringVar()
plusLabel.set("+")
plusLabel = Label(frame1,textvariable=plusLabel)
plusLabel.grid(row=2,column=0)
lineC = Canvas(frames[3], width=600,heigh=10)
lineC.create_line(0,5,590,5, fill="black",width=1)
lineC.grid(row=0, column=0)
def calc():
values1= getValues(first)
values2= getValues(second)
t1 = calcTime(values1)
t2 = calcTime(values2)
res = t1 + t2
myValues = convertTime(res)
fillEntries(myValues,third)
return
eqButton = Button(frame1,text="=",command=calc,bg="white")
eqButton.grid(row=4,column=0)
def clearET():
for i in range(4):
first[i].delete(0,"end")
second[i].delete(0,"end")
third[i].delete(0,"end")
clButton = Button(frames[5],text="Clear",bg="skyblue",command=clearET)
clButton.grid(row=0,column=0,pady=20)
main_window.mainloop()
The Output
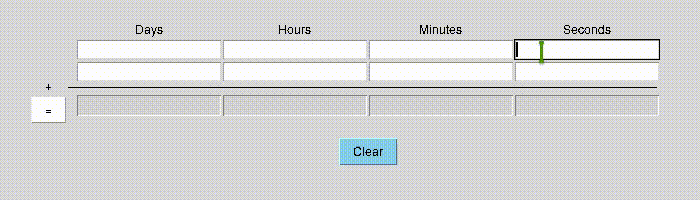
Something to say?
If you want to add something about this post, please feel free to do it by commenting below 🙂.