
The Message Widget inside a For Loop
Post by Amina Delali, January 02nd, 2023
Description
The Message widget resample the label widget, but it has one noticeable difference: the associated text is automatically wrapped. This can be useful, in case we don't know the text in advance, or if the if the may later change.
To simulate the fact that the text of the widget will be generated automatically while the program is running, we will use a for loop that will add different text lines to the widget. To demonstrate the wrapping and the automatic change of the width of the widget, we will use a button that will cause the execution of the previous loop.
And to show the difference between the message widget, and the label widget, we will apply the same changes on both the message and the label widget.
In the code below, we will:
- Create a list of 3 strings (3 elements) called lines.
- Create a message widget (myMessage), and a label widget.
-
Define two functions: addTheText and clearTheText. The addTheText function will include the for loop:
- The addTheText function will loop through the lines list, and will concatenate the previous text of the message widget with the new line. The same thing is applied on the label widget.
- The clearTheText function will clear the text of both the message and the label widget.
-
The functions will not be called unless the user click on one of these two buttons:
- buttonAT: each time the user click on that button, the addTheText function will be called.
- buttonCT: each time the user click on that button, the clearTheText function will be called.
The Code
from tkinter import *
main_window=Tk()
main_window.title("Message inside a Loop")
main_window.geometry('500x300+400+100')
main_window.configure(bg="#8800FF")
lines =["This is the beginning of the text."," It is going to be automatically wrapped,"," and you are going to see how."]
myLabel = Label(main_window,text="")
myLabel.place(relx=0.02, rely=0.75)
myMessage = Message(main_window, text = "",bg="#f4FF00",
font=("Constantia",12,"normal"))
myMessage.place(relx=0.5,rely=0.5, anchor="center")
def addTheText():
for line in lines:
myMessage ["text"] = myMessage ["text"] + line
myLabel["text"] = myLabel["text"] + line
def clearTheText():
myMessage ["text"] = ""
myLabel["text"] = ""
buttonAT = Button(main_window,text="Add the Text",width=10,
bg="#0077FF", fg="white", command=addTheText)
buttonAT.place(relx=0.8,rely=0.1,anchor="w")
buttonCT = Button(main_window,text="Clear",width=10,
bg="#0077FF",fg="white", command=clearTheText)
buttonCT.place(relx=0.8,rely=0.2,anchor="w")
main_window.mainloop()
The Difference between the Message and the Label Widget
The difference between the Message widget and the Label widget in Python Tkinter, is that in the Message widget, the text is automatically wrapped, and if the text changes, the width of the widget will also be changed accordingly (to preserve the aspect ratio). And in the label widget, the text is not automatically wrapped, but you can set the wraplength attribute to the maximum number of characters that you want to be displayed in each line.
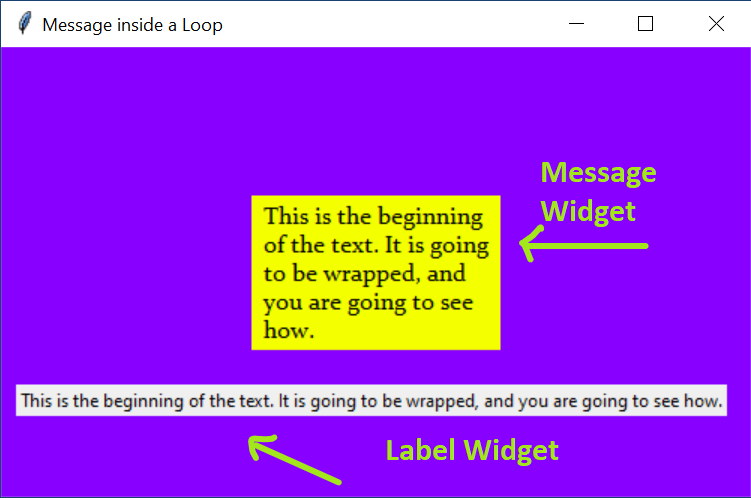
Something to say?
If you want to add something about this post, please feel free to do it by commenting below 🙂.